Content Browser SDK (V1)
Version 1 of the Content Browser SDK allows users to find and select images and assets that belong to the "Other" Asset Type.
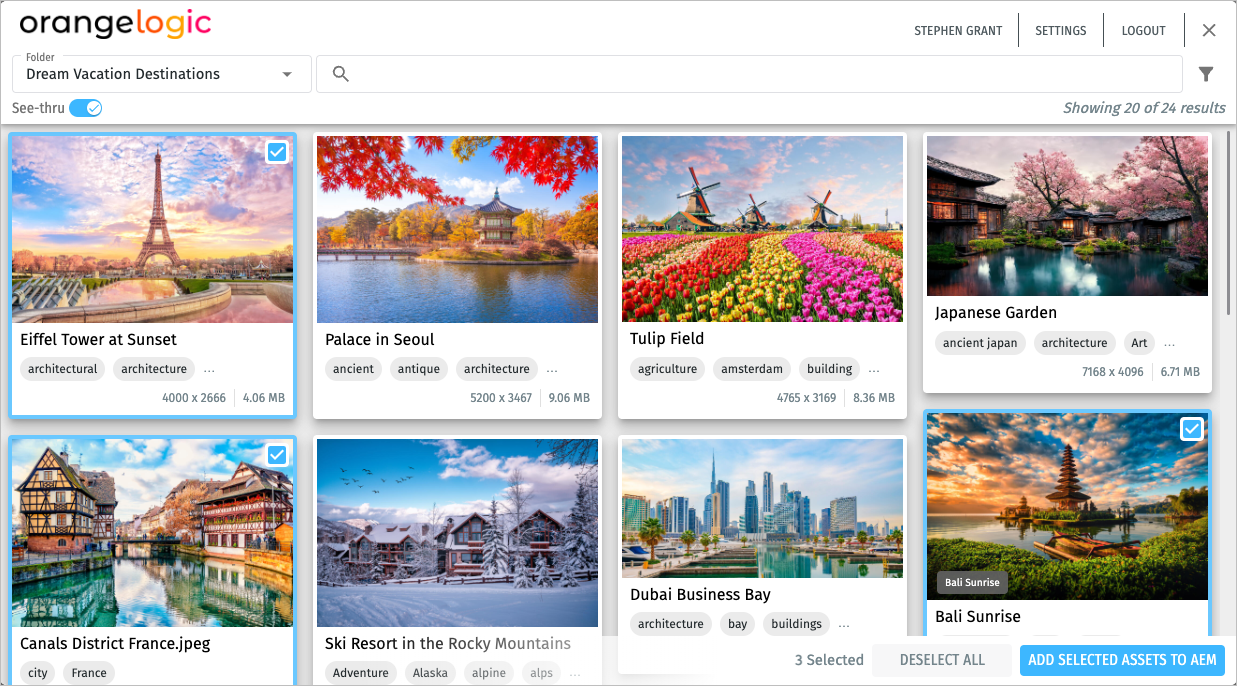
Sample Content Browser (V1)
Note
- The Content Browser SDK used to be called the “Generic Asset Browser.”
- If your organization uses Orange Logic Milan or later, we recommend using version 2 of the Content Browser SDK.
Add the Content Browser to your Orange Logic environment
There are two ways to add the content browser to your Orange Logic environment:
- Download the content browser and install it in your Orange Logic environment.
- Access the content browser via Orange Logic’s CDN.
After you add the content browser to your environment, you must activate IIIF in your Orange Logic environment. This allows users to view images.
Download and install the content browser
Content browser versions are separate from Orange Logic releases. Download the most content browser version that works with the Orange Logic release your organization uses.
Then, open the files while you’re signed in to Orange Logic to install the content browser on your Orange Logic environment.
Notes
- When you click the links to download the content browser, they might open in your browser instead of downloading to your computer. If this happens, you can download the file by pressing Cmd + S (Mac) or Ctrl + S (Windows) to save each file to your computer.
Change Log
Version | Date available | Status | Major changes | Minor changes | Compatible Orange Logic releases |
---|---|---|---|---|---|
1.2.3 (.js ; .css ) | Not supported | Milan and later | |||
1.2.2 (.js ; .css ) | December 20, 2024 | Not supported | Updated the download link structure. | Milan and later | |
1.2.1 (.js ; .css ) | November 8, 2024 | Not supported | Resolved a CSS conflict with Adobe Experience Manager. | Irvine and later | |
1.2.0 (.js ; .css ) | July 09, 2024 | Not supported | The content browser now displays encrypted asset thumbnails. | Irvine and later | |
1.1.0 (.js ; .css ) | May 29, 2024 | Not supported |
| Irvine and later | |
1.0 (.js ; .css ) | Not supported | Irvine and later |
Activate IIIF in your Orange Logic environment
Orange Logic’s content browser displays images following International Image Interoperability Framework (IIIF) standards. These standards allow images shared with IIIF to be opened with image viewers. To use the content browser, you or an administrator must activate IIIF support in your organization’s Orange Logic environment and choose which folders and images should be available in image viewers. For more information, go to the IIIF support article.
Security Function
You must add the Can use IIIF API Security Function to the *Everyone Group. This allows anyone to view images shared with IIIF in the content browser and other image viewers.
Add the Content Browser SDK (V1) to a project
You can add the content browser to projects created with HTML, JavaScript, React, and more. These instructions explain how to install the content browser with HTML. Go to the example section for examples created with JavaScript and React.
Follow these steps to access the content browser via a Cloud Delivery Network (CDN) in a webpage.
-
Paste the following HTML into your webpage.
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8" /> <meta name="viewport" content="width=device-width, initial-scale=1.0" /> <link rel="stylesheet" href="https://fonts.googleapis.com/css?family=Roboto:300,400,500,700&display=swap" /> <script src="{Content-Browser-SDK-js-download-link}"></script> <link rel="stylesheet" type="text/css¨" href="{Content-Browser-SDK-css-download-link}"> </head> <body> <div style="display:flex; flex-direction: column; gap: 0.5em;" id="app"> <span>containerId: "react-app-root". </span> <div style="display:flex; gap: 0.5em;"> </span>Try CortexAssetPicker.help() in the console or click this button</span> <button style="width: 250px; height:20px;" onclick="myFunction()">Load the Content Browser</button> </div> <div id="react-app-root" style="width: 800px; height:800px; border: 2px solid black;"></div> </div> <script> function myFunction() { CortexAssetPicker.open({ onImageSelected: function (assets) { console.log(assets); }, onError: function (error) { console.log(error); }, containerId: "react-app-root", baseUrl: "<your-organization's-Orange-Logic-URL>", }); } </script> </body> </html>
-
(Optional) In the line beginning with
baseUrl
, replace<your-organization's-Orange-Logic-URL>
with your organization’s base Orange Logic URL. For example,https://mangovations.orangelogic.com/
. -
In the line beginning with
<script
, replace{Content-Browser-SDK-js-download-link}
with the .js download link from the latest release. -
In the line beginning with
<link
, replace{Content-Browser-SDK-css-download-link}
with the .css download link from the latest release. -
To confirm the CDN loaded, call
CortexAssetPicker.help()
in the web console. This will return:/* Cortex Asset Picker Help */ window.CortexAssetPicker.open({ onImageSelected: (images) => { // Do something with image console.log(images); }, onError: (errorMessage, error) => { // Do something with error }, multiSelect: true, // single or multiple file picker containerId: "react-app-root", // container element to attach, leave blank to open popup extraFields: ["coreField.OriginalFileName", "document.CortexPath"], // extra asset fields to get baseUrl: "<your-organization's-Orange-Logic-URL>", // default base URL to pre-filled; onlyIIIFPrefix: true, // default is false will get full IIIF standard file link displayInfo: { title: true, dimension: true, fileSize: true, tags: true,} });
Configure the content browser
You can customize the content browser to fit your use case:
Customize the content browser on your own
Open the content browser with the following function:
window.CortexAssetPicker.open(image) => {
console.log(image)
}
Then, pass configurations to content browser and control its behavior by adjusting these attributes:
Attribute | Description | Required | Default value | Possible values |
---|---|---|---|---|
multiSelect | When true , users can select more than one asset at a time. | no | false | true , false |
containerId | The container ID that the content browser attaches to. If undefined, the content browser appears as a popup. | no | null | string |
baseUrl | If specified, the content browser prefills the base URL as the specified value. Otherwise, the URL on the login page will be empty. | no | null | URL string |
onlyIIIFPrefix | IIIF links have this format:{scheme}://{server}{/prefix}/{identifier}/{region}/{size}/{rotation}/{quality}.{format} When false , the link of an IIIF image is the full URL, for example:{scheme}://{your-Orange-Logic-server}/IIIF3/Image/2Y1XC5O9J67/full/max/0.0/default.jpg When true , the link of an IIIF image is shortened to the URL before the {region} , for example:{scheme}://{your-Orange-Logic-server}/IIIF3/Image/2Y1XC5O9J67 | no | false | true , false |
displayInfo | Specifies whether the Image Asset card displays information for these fields: title, dimension, fileSize, and tags. Use true to show a field and false to hide it. | no | { title: true, dimension: true, fileSize: true, tags: true, } | ImageCardDisplayInfo { title: <true, false>, dimension: <true, false>, fileSize: <true, false>, tags: <true, false>, } |
onImageSelected | The information returned when one or more images is selected. This takes a callback function, and once the user selects assets, this information is returned: ImageLink: The asset’s link Metadata: Select metadata for each asset, configured in the server that has the assets. Orange Logic configures this metadata for you. ExtraFields: Fields requested by the user | yes | null | (image) => { console.log(image) } |
onError | The callback when an error occurs. The end user will have access to the Error message and the Error object. | no | null | (errorMessage, error) => { console.log(errorMessage) } |
extraFields | You can request extra Orange Logic metadata fields via this attribute. Use Orange Logic API names separated by commas. | no | null | For example: ["CoreField.OriginalFileName",”CoreField.CortexPath"] |
Customizations Orange Logic can make
Submit a support request to do any of the following:
- Limit asset availability based on visibility class. For example, Orange Logic can configure the content browser to display only images with the Visibility Class “Public.”
- Change the structure of asset URLs. For example, you could use IIIF image adjustments. Go to the IIIF structure for the default structure.
- Configure the returned metadata. You can choose which metadata is returned with the
Metadata
sub-field of the attributeOnImageSelected
. - Change the returned asset format. You can choose which proxy formats are available to your users. Then, they can choose the format they want when they select an asset.
Note
In v1.0.0 of the Content Browser SDK, users cannot select the proxy to use. Instead, users access the large proxy format unless you choose another proxy format as the default.
IIIF structure
Orange Logic supports IIIF Image API 3.0 standards, so by default you can use the following syntax to retrieve an image:
[SITE_URL]/IIIF3/Image/[Identifier]/[Region]/[Size]/[Rotation]/[Quality].[Format]
where [Identifier]
is the selected asset’s Orange Logic RecordID. This is the encrypted version of the SystemID.
Go to the IIIF support article for more information.
Use the content browser with other APIs
The content browser can return the Orange Logic SystemID or RecordID of selected assets. Then, you can use these identifiers with Orange Logic’s APIs and tools to do even more with those assets. For example, you could update the selected asset’s metadata within Orange Logic to reflect how it is used in the external application.
Content browser examples
The content browser is extremely flexible. Use these examples as inspiration.
Orange Logic’s demo page
You can try the content browser for yourself on our demo page.
Note
The version 1 demo only works if your organization has activated the content browser on your Orange Logic site. To learn how to activate the content browser on your site, go here.
JavaScript
In this example, the extraField
attribute is specified as ["CoreField.alternative-description"]
and onImageSelected
is specified as:
function (assets) {
console.log(assets);
document.getElementById("myImage").src=assets[0].imageUrl;
document.getElementById("myImage").alt=assets[0].extraFields['CoreField.alternative-description'];
}
Therefore, the selected image’s alternative description is displayed when a user hovers over it.
React
Sanity.io is a CMS that stores and pushes content to digital platforms. Orange Logic’s Sanity.io integration retrieves images from Orange Logic and adds them to Sanity. It’s a great example of using Orange Logic’s Content Browser SDK in an integration.
Updated 2 months ago